How To Write A Blackjack Program In C
Now I’d like to share one of my experience of programming C.
Our teacher gave us a task: to finish a small but not so useless program by our own in C. He gave three options and unluckily, I was so confident that I chose the most difficult one: to write a program of Blackjack (simplified though, without money involved), also known as Twenty-one, played in command line.
It is a disaster. Though I scanned lots of programming books, however, I haven’t really finished a useful project before. In this task, I strongly realize how stupid I am.
You need to add the preceding space in the scanf statement, like so: '%c'. This will consume the newline character for you. One clue as to solving this is actually already in your code! The two getchar calls at the end are serving this very purpose: once the program finishes, wait for the user to hit Enter. However, you required two getchars. A blackjack program I wrote in C, it's kind of awful - blackjack.cpp. Write a function that takes the values of a two-card blackjack hand as input, and prints out the point total of the hand. The value of the cards '2' through '9' is equal to their face value, the cards 'T', 'K', 'Q', 'J' are worth 10 points and the ace ('A') is worth 11 points unless it comes with another ace, then it's worth 1 point. The program should be able to catch incorrect input.
First of all, I don’t know the way to shuffle the 52 cards. Their order requires random, no repeat, and easy to access. “Array”, you must say. However, I didn’t exactly understand how it works in C, and I had to find out the algorithm, which our teacher didn’t tell us. And after I found the answer to the algorithm, I was trapped in the pointers and arrays, and my poor understanding to function in C forced me to face strange warnings and errors. I wasted long long time to debug the shuffle_cards() function, though in the end I realized how simple it is.
Secondly, too many branches in the game exactly annoyed me a lot. There are too many possible results of the game, due to its rules. So I had to use lots of if(){}else{} statement, which results in ugly look and slow performance.
Finally, problems occur when I want to compile the code. I develop it in Ubuntu Linux, and gcc works fine. But it seems that Visual C++ 6.0 doesn’t like the code! Luckily I found alternative compiler in Windows: Dev C++ and Tiny C Compiler. And I finished it now. They are ugly and unclean codes. :-(

It is an unforgettable experience. Well, anyway, after all, I dare to say I have developed in C now!
I have uploaded it to https://github.com/fyears/simple-Black-Jack now.
Related Posts
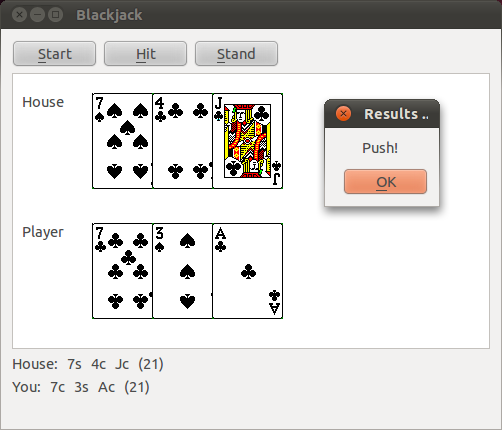
The following tutorial is only some kind of “thought provoking” one: shows you some logic and class structure using enums and dynamically created images with Winform application.
Maybe I skipped some of the official rules. If so, I’m sorry I’m not a big gambler. This tutorial is not for teaching you Blackjack, but showing you some C# practices.
Here are some of the rules I have implemented:
- we have a shuffled deck of 52 cards
- the player starting hand has 2 cards
- the computer hand has also 2 cards: one of them is visible the other one is not
- the Jack, Queen and King has the value of 10
- the Ace has the value of 11 or 1 if 11 would be too much in hand
- the player starts to draw (official phrase is HIT)
- the main goal is to stop at 21 (or close to 21)
- the player can skip the drawing phase (STAY) if the total score is 16 at least
- if player stays, the computer turn comes
- first we can see the computer’s second (hidden) card
- the computer starts to draw using the same rules as ours mentioned before
To show you how to use System.Drawing.Graphics class, I create the images of the cards by cutting 1 big image into pieces. Click on the following image to download it:
I have 3 main classes: Card, Deck and Hand. Of course the form has its own Form1 class.
I also have 2 enums: CardValue and CardSuit. Both enum indexing starts from 1. The CardValue contains the type of the cards (Ace, King, 9, 7, 2, etc.). The CardSuit contains the colors (hearts, spades, etc.).
Create a new class: Card.cs
Under the class place the 2 enums:
The Card class will have 3 properties, 1 constructor, 1 new method and 1 overridden method. What properties does a card have? Of course its value, its color and its picture. So create the private properties with their public encapsulated fields. The Image property won’t have setter, the value and color setter will have the image loader method:
With the constructor let’s create a dummy card with no color and value:

The attached image has the following properties: it’s 392 pixel high and 950 wide. So 1 card is about 97 high and 73 wide. The method has to slice the image depending on the following color from the deck (one color in each row on the image) and depending on the value (the image has the values in ascending order).
How To Write A Blackjack Program In Computer
And the overridden ToString method, just for fun (not used later in the code):
How To Write A Blackjack Program In C Programming
In the next chapter I’ll show you how to create the class for the Hand and the Deck.